Loops are one of the most useful tools in programming, allowing you to repeat actions multiple times. In this guide, we’ll explore two types of loops in Java: while
and do-while
. These loops are similar but have key differences that make them suitable for different tasks.
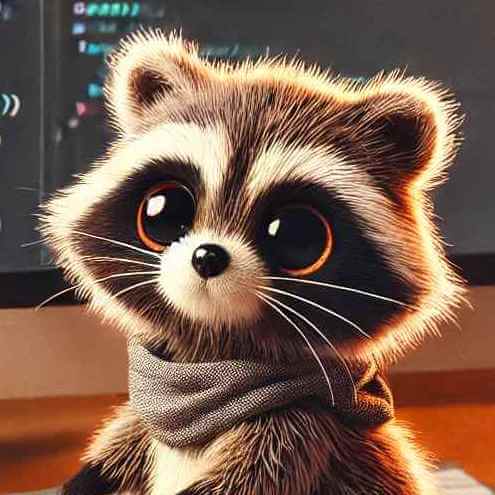
What Is a Loop, and Why Do We Need It?
A loop is a way to tell your program to repeat an action as long as a specific condition is met. Loops are handy in situations like:
- Asking a user to re-enter input until it’s valid.
- Processing large datasets.
- Performing tasks when the number of repetitions isn’t known beforehand.
Imagine this: you want your friend to pour water into a glass 50ml at a time until the glass is full. A loop is like giving instructions for this process.
The while
Loop
The while
loop checks the condition first, and only if it’s true, it performs the actions inside the loop. If the condition is false from the start, the loop won’t run at all.
Example: Filling a Glass of Water
int waterInGlass = 0; // Water in the glass (0 ml)
int fullGlass = 250; // Full glass (250 ml)
while (waterInGlass < fullGlass) {
waterInGlass += 50; // Add 50 ml of water
System.out.println("The glass now contains " + waterInGlass + " ml of water.");
}
System.out.println("The glass is full!");
How It Works:
- The loop keeps running as long as the amount of water in the glass is less than 250 ml (
waterInGlass < fullGlass
). - Each iteration adds 50 ml of water to the glass.
- Once the glass is full, the loop stops.
The do-while
Loop
The do-while
loop is a bit different. It performs the actions first and then checks the condition. This means the loop will run at least once, even if the condition is false from the start.
Example: Printing a Message Once
int count = 6;
do {
System.out.println("This message will be printed at least once.");
count++;
} while (count < 5);
What Happens Here:
- The variable
count
starts at 6, so the conditioncount < 5
is false. - However, because the
do-while
loop executes the actions before checking the condition, the message is printed once before the loop ends.
When to Use while
and do-while
Loops
while
: Use this loop when you want actions to run only if the condition is true.do-while
: Use this loop when the actions should run at least once, regardless of the condition.
Handy Features for Loops
break
— Exit the Loop Early:
If you need to stop the loop before it naturally ends, usebreak
.
int count = 0;
while (true) {
System.out.println("Iteration " + count);
count++;
if (count == 5) {
break; // Exit the loop when count reaches 5
}
}
continue
— Skip to the Next Iteration:
Usecontinue
to skip the rest of the code in the current loop iteration and jump to the next one.
int count = 0;
while (count < 10) {
count++;
if (count % 2 == 0) continue; // Skip even numbers
System.out.println("Odd number: " + count);
}
Comparing while
and do-while
Loops
Feature | while | do-while |
---|---|---|
Condition Check | Before executing the loop body | After executing the loop body |
Runs Without Condition | No, if the condition is false | Yes, at least once |
Example Use Case | Repeating until valid user input | Displaying a menu before checking the exit condition |
Real-Life Examples of Loops
- Ask for User Input Until It’s Correct:
import java.util.Scanner;
Scanner scanner = new Scanner(System.in);
String correctPassword = "java123";
String userInput = "";
while (!userInput.equals(correctPassword)) {
System.out.println("Enter the password:");
userInput = scanner.nextLine();
}
System.out.println("Correct password! Access granted.");
- Sum Numbers Until the User Quits:
import java.util.Scanner;
Scanner scanner = new Scanner(System.in);
int sum = 0;
do {
System.out.println("Enter a number (or 'q' to quit):");
String input = scanner.nextLine();
if (input.equals("q")) break;
sum += Integer.parseInt(input);
} while (true);
System.out.println("Total sum: " + sum);
Tips for Working with Loops
- Avoid Infinite Loops:
Always make sure the loop has a way to stop, or it will run forever.Example of an infinite loop:
while (true) {
System.out.println("This is an infinite loop!");
}
- Use
break
andcontinue
Wisely:- These commands let you control the flow of the loop more precisely.
- Pick the Right Loop:
- Use
while
when the condition must be true for the actions to run. - Use
do-while
when you need the actions to run at least once.
- Use
Summing It Up
Loops like while
and do-while
make your programs more efficient by automating repetitive tasks.
while
is great for situations where you need to check the condition first.do-while
works well when at least one execution of the actions is guaranteed.
Practice writing loops in Java to see how they can simplify your coding tasks. Happy coding!