Java Junior Interview Questions and Answers
/**
* There are tons of pages out there with similar content.
* Ours is going to be just as good, not better or worse.
* Don’t think you have to memorize all the answers before you go to an interview.
* This page is more like a beginner programmer’s go-to guide.
* You should read a few sections every day.
* That’s exactly how we’ll keep updating the content on this page.
*
* The text of the questions is clickable.
*/
1. Primitive data types.
In Java, there are several primitive data types that are used to store simple values. Here’s a quick rundown:
- int: This is used to store integer numbers (whole numbers) like -10, 0, 42, etc.
- double: This is used to store floating-point numbers (numbers with decimal points) like 3.14, -0.5, 100.0, etc.
- boolean: This is used to store true or false values, representing binary logic.
- char: This is used to store a single character, like ‘a’, ‘7’, ‘$’, etc.
- byte: This is used to store small integer numbers within the range of -128 to 127.
- short: This is used to store slightly larger integer numbers within the range of -32,768 to 32,767.
- long: This is used to store very large integer numbers within the range of -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
- float: This is used to store floating-point numbers with less precision compared to double.
These primitive data types are the building blocks for storing data in Java programs. Each type has its own range of values that it can store, which you need to keep in mind when working with them in your
2. How to correctly set a variable identifier in Java?
In Java, choosing the right identifier for a variable involves following certain rules and best practices, especially for beginners. Here’s a straightforward guide:
- Start with a Letter: Identifiers must begin with a letter (A-Z or a-z), currency character (like
$
), or an underscore (_
). However, starting with a letter is usually preferred for readability. - No Spaces Allowed: Identifiers cannot contain spaces. If you need to separate words in an identifier, use camelCase (e.g.,
myVariable
) or underscores (e.g.,my_variable
). - Case Sensitive: Java is case sensitive, which means identifiers like
myvariable
,myVariable
, andMYVARIABLE
are considered different. - Avoid Reserved Words: Java has reserved words that you cannot use as identifiers, such as
int
,class
, orvoid
. These words are part of the language syntax. - Meaningful Names: Choose names that clearly describe what the variable represents. For example, use
studentCount
instead of vague names likesc
orcount
. - Length: There is no limit to the length of an identifier, but it should be concise yet descriptive.
Here’s an example of declaring some well-named variables in Java:
int itemCount = 10;
String userName = "JohnDoe";
boolean isGameOver = false;
These guidelines help maintain clean, readable code, which is crucial for both learning and collaborative programming environments.
3. How many keywords are reserved by the language, what are these words, which ones are not used?
50, two of them are not used: const, goto;
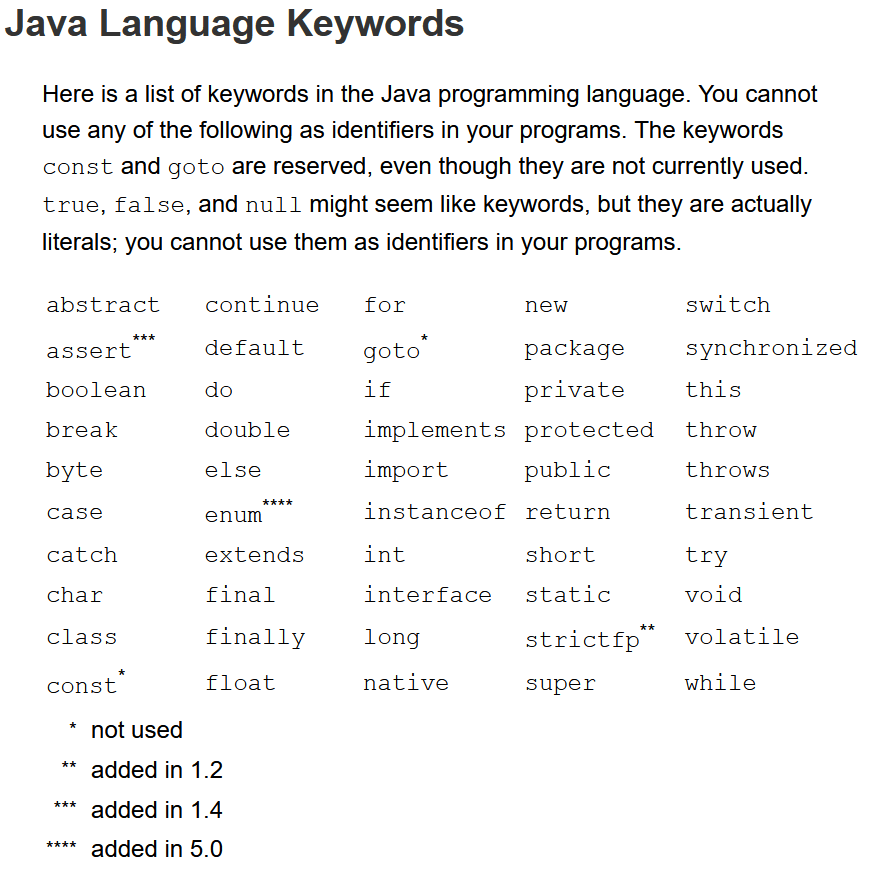
4. Java Keywords Categorized
Data types:
byte, short, int, long, char, float, double, boolean
enum;
Loops and branches:
if, else, switch, case, default, while, do, break, continue, for;
Exceptions:
try, catch, finally, throw, throws;
Access Modifiers:
private, protected, public;
Declaration, Import:
import, package, class, interface, extends, implements, static, final, void, abstract, native;
Creat, Return, Call:
new, return, this, super;
Multithreading:
synchronized, volatile;
Other:
instanceof, assert, transient, strictfp, const, goto
5. Variable initialization
In Java, initializing a variable means assigning an initial value to the variable.
Here are examples of variable initialization in Java:
Initialization of primitive data types:
java
int number = 10;
char letter = 'a';
boolean isJavaFun = true;
Initialization of object data types (classes and arrays):
java
String greeting = "Hello, world!"; int[] numbers = {1, 2, 3};
Initialization can occur immediately when declaring a variable or separately:
java
int age; // Declaration
age = 30; // Initialization
Additionally, Java allows for variable initialization through constructs such as class constructors or methods if dealing with more complex data types or custom objects.
- Declaration:
- This is when you specify the type of an object and its name but do not create an instance of it. At this stage, no memory for the object itself is allocated; only a reference of type
MyClass
is created, and it does not point to any actual object. It’s equivalent to holding an empty box that is labeled to hold an object of typeMyClass
.
- This is when you specify the type of an object and its name but do not create an instance of it. At this stage, no memory for the object itself is allocated; only a reference of type
- Initialization:
- This involves actually creating an instance of the class (allocating memory) using the
new
keyword followed by the constructor of the class. This not only allocates the memory but also sets up the initial state of the object as defined by the constructor.
- This involves actually creating an instance of the class (allocating memory) using the
Here’s a Java code example illustrating both scenarios:
java
// Declaration of a variable to hold an object of type MyClass
MyClass myClass;
// Initialization of the variable 'myClass' to point to a new instance of MyClass
myClass = new MyClass();
In this example:
- The line
MyClass myClass;
is where the declaration happens. At this point,myClass
is just a reference and doesn’t point to an actual object. - The line
myClass = new MyClass();
is where the initialization occurs. Here, memory is allocated for a new object of typeMyClass
, and the referencemyClass
is updated to point to this new object.
Without initialization — ” = “, Java simply reserves the name, the variable is declared to be the specified type.
6. What main groups can data types be divided into?
In Java, data types can be broadly divided into two main groups:
7. Is Java Platform Independent if then how?
Yes, Java is widely recognized as a platform-independent programming language. This characteristic is often summed up in the popular Java slogan: “Write Once, Run Anywhere” (WORA). This means that once you write your Java code and compile it, the resulting bytecode can run on any device that has a Java Virtual Machine (JVM), regardless of the underlying operating system or hardware.
Here’s a simple explanation:
1. Java Code to Bytecode
- When you write a Java program, you write it in plain text files ending with the
.java
extension. This code is in high-level Java language that you, as a programmer, can understand and write. - You then compile this code using the Java compiler (
javac
). The compiler checks your code for errors and, if no errors are found, converts your high-level code into bytecode. Bytecode is the intermediate representation of your code that is neither high-level code nor machine code (binary code specific to a particular type of processor).
2. Bytecode to Machine Code
- The bytecode generated by the Java compiler is not specific to any one machine. Instead, it’s a generic code that can be run on any machine that has a JVM.
- When you run the Java program, the JVM on the device takes this bytecode and converts it into machine code through a process called Just-In-Time (JIT) compilation or interprets the bytecode directly. This machine code is what the computer actually understands and can execute.
3. Java Virtual Machine (JVM)
- The JVM is the cornerstone that makes Java platform-independent. Each operating system (Windows, macOS, Linux, etc.) has its own JVM that is designed to work specifically with its underlying system architecture and operating system features.
- The JVM handles the details of converting bytecode into machine-specific code and interacting with the system hardware and resources. This abstraction allows Java bytecode to be platform-independent, as the same bytecode can be run on any JVM, regardless of the underlying platform.
Summary
To sum it up, the platform independence of Java comes from the fact that its programs are compiled into a universal bytecode format, which any JVM can interpret or compile into native machine code on the fly. This makes distributing Java applications easy and universal across different systems, as the same bytecode can run on any machine that has a compatible JVM.
8. Conversion of primitive data types, is there any loss of data, is it possible to convert a Boolean type.
In Java, primitive data type conversions can occur in two ways: implicit (automatic) conversion and explicit conversion. Here’s a brief explanation of each, including information about data loss and the possibility of converting a boolean type.
Implicit Conversion
Implicit (or automatic) conversion happens when a smaller data type is automatically converted to a larger type without loss of information. For example:
byte
toshort
,int
,long
,float
, ordouble
short
toint
,long
,float
, ordouble
char
toint
,long
,float
, ordouble
int
tolong
,float
, ordouble
long
tofloat
ordouble
float
todouble
This type of conversion is safe in terms of precision or data volume loss.
Explicit Conversion
Explicit conversion (or type casting) is required when a larger data type is cast to a smaller type. This is done using a casting operator. For example, if you convert a double
to an int
, you must explicitly indicate this:
java
double d = 9.97;
int i = (int) d; // i will be 9, losing the fractional part
In this case, data loss is possible, as floating-point types may lose their fractional parts, and large numbers may be truncated to fit the range of the target type.
Conversion of Boolean Type
The boolean data type in Java cannot be converted to any other type or from any other type. Boolean values true
and false
do not have a numerical representation, unlike some other programming languages where, for example, true
might be equivalent to 1
, and false
to 0
. In Java, such conversions are not supported:
java
boolean b = true;
// int i = (int) b; // Compilation error
Conclusion
It’s important to understand the differences between implicit and explicit conversions to avoid unexpected data loss, especially when working with floating-point types and large integers. Conversion from or to boolean
is not supported in Java.
9. What is the difference between “fail-fast” and “fail-safe” iterators in Java?
In Java, the concepts of “fail-fast” and “fail-safe” iterators are distinguished, often translated as “fail-fast” and “fail-safe” respectively. These terms describe the behavior of iterators in a multi-threaded environment and when modifying a collection during iteration. Let’s consider each type:
- Fail-fast iterators:
- Fail-fast iterators are designed to quickly detect errors that arise due to modifications of the collection during iteration, except when modifications are made through the iterator itself (e.g.,
remove()
,add()
methods of the iterator). - If the collection is modified anywhere in the program, not using iterator methods, after the iterator is created and before the iteration cycle is completed, the iterator will “fail fast” by throwing a
ConcurrentModificationException
. - Examples of such iterators in Java include iterators from most standard implementations of collections in the
java.util
package (e.g.,ArrayList
,HashMap
).
- Fail-fast iterators are designed to quickly detect errors that arise due to modifications of the collection during iteration, except when modifications are made through the iterator itself (e.g.,
- Fail-safe iterators:
- Fail-safe iterators do not throw a
ConcurrentModificationException
if the collection is modified during iteration. They operate on copies of the original data, allowing the collection to change without affecting the iteration process. - These iterators may potentially work with outdated data if the collection was modified during the iteration.
- Examples of such iterators can be found in packages that use concurrent collections, such as
java.util.concurrent
(e.g.,CopyOnWriteArrayList
,ConcurrentHashMap
).
- Fail-safe iterators do not throw a
The choice between “fail-fast” and “fail-safe” depends on the specific requirements of the application and whether performance or reliability is more important when working with collections in a multi-threaded environment.
10. What are exceptions in Java?
Exceptions are unwanted or unexpected events, which occur during the execution of a program i.e., at runtime, that disrupts the normal flow of the program’s instructions.
11. What is the main method in Java?
As a junior developer, it’s important to understand the main method in Java as it serves as the entry point for any standalone Java application. This is the method where your program begins execution.
The Structure of the Main Method
The main method in Java has a very specific signature that you need to follow for your application to run:
java
public static void main(String[] args) {
// Your code goes here
}
Breakdown of the Main Method Components
- public: This access modifier allows the main method to be accessible from anywhere. It’s crucial because when you run your program, the JVM (Java Virtual Machine) needs to access this method without any restrictions.
- static: Since static methods can be called without creating an instance of the class, the
static
keyword ensures that the JVM can invoke the main method without needing to instantiate its class. This is essential because at the start of your program, no objects are instantiated yet. - void: This specifies that the main method does not return any value. It’s meant solely to start the program.
- main: The name of the method that the JVM looks for as the starting point of the program. It must be spelled exactly as “main”.
- String[] args: The parameter passed to the main method is an array of Strings, which allows users to pass arguments to the program from the command line or any other environment from which the program is started. These are optional to use within your program but must be included in the method signature.
Mandatory Conditions for the Main Method
To serve as the entry point of a Java application, the method must adhere to these rules:
- It must be declared
public
. - It must be declared
static
. - It must not return any value (
void
). - It must be named
main
. - It must accept a single parameter: an array of
String
values (String[] args
).
There can be several main methods. If this method is not present, then compilation is possible, but at startup there will be an Error: Main method not found.
12. What is ClassLoader and what is it for?
In Java, the ClassLoader
plays a crucial role in the dynamic loading of classes during the runtime of an application. Here are the key functions of a ClassLoader
:
- Class Loading: The
ClassLoader
loads classes into the Java Runtime Environment (JRE) when they are needed by the program but not yet present in memory. This allows Java applications to be modular, loading only the necessary resources, which is particularly important in large or complex applications. - Namespace Management: Each
ClassLoader
creates its own namespace. This means that two classes with the same name can coexist if they are loaded by differentClassLoader
s, which is important for application isolation in environments like application servers. - Security: The
ClassLoader
can verify the bytecode of classes for compliance with specific security criteria before the classes are used in the program. This can include checking digital signatures or ensuring compliance with security policies. - Delegation: Java often uses a delegating model for class loading. When a
ClassLoader
receives a request to load a class, it first delegates the request to its parentClassLoader
. Only if the parent loader cannot find the class does the childClassLoader
attempt to load the class itself. This ensures a class loading order that helps prevent conflicts. - Flexibility: Users and developers can create their own
ClassLoader
to extend or modify the standard class loading behavior. This can be used for dynamically loading classes from non-standard sources, such as network resources or archive files.
Overall, the ClassLoader
in Java provides a powerful tool for managing the loading and isolation of classes in the runtime environment, supporting modularity, security, and extensibility of applications.
13. What logical operators exist in Java?
What is the difference between the short and full notation of logical operators?
Logical comparison operations: >, <, <=, >=, ==, !=;
Other logical operators (besides comparison operators) are usually called logical operators. These operators work with boolean values (true
and false
) and are used to perform logical operations such as conjunction, negation, and other logical operations.
>
— greater than<
— less than>=
— greater than or equal to<=
— less than or equal to==
— equal to!=
— not equal to
Logical Operators:?:
— ternary conditional operator
(example: condition ? value_if_true : value_if_false
)&&
— logical AND
(short-circuit AND: checks the second operand only if the first is true)||
— logical OR
(short-circuit OR: checks the second operand only if the first is false)!
— logical NOT
If both operands are true, then the & and && operators return true.
If at least one operand is true, then the | and || operators return true.
The operators & and | always evaluate the value of both operands (full). && and || are called short-circuit operators because if the result of the boolean expression can be determined from the left operand, the right operand is not evaluated.
Note: || and && can only be used in logical expressions.
14. What is a truth table?
A truth table is a table that describes a logical function.
In the context of Java, or any programming language, you can use truth tables to understand and manage the results of logical operations, especially when making decisions or controlling flow with conditions. Here’s a basic rundown of truth tables for the standard logical operators:
1. Logical AND (&&)
The logical AND operation results in true
only if both operands are true.
A | B | A && B |
---|---|---|
true | true | true |
true | false | false |
false | true | false |
false | false | false |
2. Logical OR (||)
The logical OR operation results in true
if at least one of the operands is true.
A | B | A || B |
---|---|---|
true | true | true |
true | false | true |
false | true | true |
false | false | false |
3. Logical NOT (!)
The logical NOT operation inverts the truth value of its operand.
A | !A |
---|---|
true | false |
false | true |
In Java, you can use these logical operations to control the execution flow with if-else statements, while loops, for loops, etc., by evaluating conditions based on these truth tables. Here’s a simple example in Java to illustrate the use of logical operators:
java
public class LogicalOperationsExample {
public static void main(String[] args) {
boolean A = true;
boolean B = false;
// Using logical AND
System.out.println("A && B: " + (A && B)); // Outputs false
// Using logical OR
System.out.println("A || B: " + (A || B)); // Outputs true
// Using logical NOT
System.out.println("!A: " + (!A)); // Outputs false
}
}
This code demonstrates how the truth values from the truth table are applied in logical expressions in Java.
15. Tell about the ternary operator.
In Java, the ternary operator is a concise way to perform conditional logic. It’s a one-liner replacement for an if-else
statement and is written as condition ? expression1 : expression2
. Here’s how it works:
- If the
condition
is true,expression1
is evaluated and its result is returned. - If the
condition
is false,expression2
is evaluated and its result is returned.
Here’s a simple example:
java
int a = 10;
int b = 5;
int max = (a > b) ? a : b; // max will be 10
In this example, the ternary operator checks if a
is greater than b
. Since it is, a
is assigned to max
. If b
were greater, b
would be assigned to max
instead.
16. What unary and binary arithmetic operations exist in Java?
In Java, arithmetic operations are a fundamental part of manipulating numbers and data. These operations can be divided into unary and binary types. Here’s a breakdown of each:
Unary Arithmetic Operations
Unary operations are those that operate on a single operand. In Java, unary arithmetic operations include:
- Unary Plus (
+
): Indicates a positive value (though it is redundant). - Unary Minus (
-
): Negates the value of the operand. - Increment (
++
): Increases the value of the operand by 1. This can be pre-increment (++x
) where the operation is performed before the value is used, or post-increment (x++
) where the value is used first and then increased. - Decrement (
--
): Decreases the value of the operand by 1. Similar to increment, this can be pre-decrement (--x
) or post-decrement (x--
).
Binary Arithmetic Operations
Binary operations involve two operands. The common binary arithmetic operations in Java are:
- Addition (
+
): Adds two operands. - Subtraction (
-
): Subtracts the second operand from the first. - Multiplication (
*
): Multiplies two operands. - Division (
/
): Divides the first operand by the second. If both operands are integers, the result is an integer division (fractional part discarded); if either operand is floating-point, the result is floating-point division. - Modulus (
%
): Computes the remainder of dividing the first operand by the second. Often used to determine if one number is divisible by another or to obtain the remainder of a division.
These operations can be used with various types of numeric data including integers, floating-point numbers, and characters (since characters in Java are stored as numbers). They form the basis of many algorithms and are integral to the operation of loops, conditions, and calculations in Java programs.
17. Java: Passing Variables (by reference/value) Explained
When you hear about “passing by value” and “passing by reference,” it might seem a bit confusing, especially in Java. Let’s clear this up in a simple way!
What Does “Passing by Value” Mean in Java?
In Java, everything is passed by value. This means when you pass a variable to a method, you’re actually passing a copy of the value stored in that variable, not the original variable itself.
- Primitive Types: For primitive types like
int
,double
,char
, etc., Java copies the actual numeric value or character value of the variable. When this value is passed to a method, any changes to this value in the method do not affect the original variable outside the method.
void addOne(int number) {
number = number + 1; // Only changes the local copy
}
int myNumber = 10;
addOne(myNumber);
System.out.println(myNumber); // Outputs 10, not 11
- Reference Types: For reference types like objects and arrays, Java copies the address (or reference) where the object is stored, not the object itself. Although it’s still passing a value (the reference – value of the reference), it feels like “passing by reference” because if you modify the object via this reference, the changes will reflect on the original object.
void changeName(Student s) {
s.name = "Alice"; // Modifies the object that 's' points to
}
Student student = new Student("Bob");
changeName(student);
System.out.println(student.name); // Outputs 'Alice'
How and Where Is the Copy Stored?
Whenever a method is called, Java creates a “stack frame” on the call stack. This stack frame holds all the local variables and parameters of the method.
- Primitive Type Copies: The actual values are stored directly in the stack frame.
- Reference Type Copies: The copied references (not the objects) are stored in the stack frame. The objects themselves live in a different memory area called the heap.
How Long Does the Copy Last?
The lifetime of the copy depends on how long the method execution lasts. When the method completes:
- Stack Frame is Cleared: Java removes the stack frame, erasing all the copies of the variables (both primitive values and references).
- Object Management: If the method had modified an object through a copied reference, the object remains in the heap until there are no more references to it or until the program ends. If no references to an object exist anymore, it becomes eligible for garbage collection (Java’s way of automatically freeing up memory).
Key Takeaways
Understanding that Java always passes by value — whether it’s the actual value for primitives or the reference value for objects — helps clarify many common confusions about how Java handles variable passing and memory management. Remember, changes to primitives inside a method won’t affect the original variables, while changes to object fields via a copied reference can alter the original object.
This explanation helps demystify how Java handles data passing, making it easier for you as a budding Java developer to predict how your programs will behave!
18. Bitwise Operations in Java
Bitwise operations are actions performed on the individual bits (0s and 1s) that make up numbers in a computer. Think of numbers as long strings of 0s and 1s. Here are the main bitwise operations in Java:
1. Bitwise AND (&
)
This operation compares each bit of two numbers. The result is 1 only where both bits are 1; otherwise, it is 0.
Example:
- Number 1:
1010
(in binary) - Number 2:
1100
(in binary)
Result of AND:
- Result:
1000
int num1 = 10; // 1010 in binary
int num2 = 12; // 1100 in binary
int result = num1 & num2; // result is 8 (1000 in binary)
2. Bitwise OR (|
)
This operation also compares each bit of two numbers. The result is 1 if at least one of the bits is 1; otherwise, it is 0.
Example:
- Number 1:
1010
- Number 2:
1100
Result of OR:
- Result:
1110
int num1 = 10; // 1010 in binary
int num2 = 12; // 1100 in binary
int result = num1 | num2; // result is 14 (1110 in binary)
3. Bitwise XOR (^
)
This operation compares each bit of two numbers. The result is 1 if only one of the bits is 1 (but not both); otherwise, it is 0.
Example:
- Number 1:
1010
- Number 2:
1100
Result of XOR:
- Result:
0110
int num1 = 10; // 1010 in binary
int num2 = 12; // 1100 in binary
int result = num1 ^ num2; // result is 6 (0110 in binary)
4. Bitwise NOT (~
)
This operation flips each bit of a number: 0 becomes 1, and 1 becomes 0.
Example:
- Number:
1010
Result of NOT:
- Result:
0101
int num = 10; // 1010 in binary
int result = ~num; // result is -11 (in 32-bit: 11111111111111111111111111110101)
5. Left Shift (<<
)
This operation shifts all bits of a number to the left by a specified number of positions, adding 0s on the right.
Example:
- Number:
1010
(shift left by 2 positions)
Result of Left Shift:
- Result:
101000
int num = 10; // 1010 in binary
int result = num << 2; // result is 40 (101000 in binary)
6. Right Shift (>>
)
This operation shifts all bits of a number to the right by a specified number of positions. The bits shifted out are lost.
Example:
- Number:
1010
(shift right by 2 positions)
Result of Right Shift:
- Result:
0010
int num = 10; // 1010 in binary
int result = num >> 2; // result is 2 (0010 in binary)
7. Unsigned Right Shift (>>>
)
This operation shifts all bits of a number to the right by a specified number of positions, filling the leftmost bits with 0s.
Example:
public class UnsignedRightShiftExample { public static void main(String[] args) { int num = -8; // In binary: 11111111111111111111111111111000 (32-bit representation) int shifted = num >>> 3; // Shift bits to the right by 3 positions System.out.println("num: " + num + " (" + Integer.toBinaryString(num) + ")"); System.out.println("shifted: " + shifted + " (" + Integer.toBinaryString(shifted) + ")"); } }
Explanation:
- Original number
-8
in binary (32-bit):11111111111111111111111111111000
- After unsigned right shift by 3 positions:
00011111111111111111111111111111
- In decimal:
536870911
Assignment Operators with Bitwise Operations
Java also has assignment operators that combine bitwise operations with assignment. Here are some examples:
AND Assignment (&=
)
int num1 = 10; // 1010 in binary
int num2 = 12; // 1100 in binary
num1 &= num2; // num1 is now 8 (1000 in binary)
OR Assignment (|=
)
int num1 = 10; // 1010 in binary
int num2 = 12; // 1100 in binary
num1 |= num2; // num1 is now 14 (1110 in binary)
XOR Assignment (^=
)
int num1 = 10; // 1010 in binary
int num2 = 12; // 1100 in binary
num1 ^= num2; // num1 is now 6 (0110 in binary)
Left Shift Assignment (<<=
)
int num = 10; // 1010 in binary
num <<= 2; // num is now 40 (101000 in binary)
Right Shift Assignment (>>=
)
int num = 10; // 1010 in binary
num >>= 2; // num is now 2 (0010 in binary)
Unsigned Right Shift Assignment (>>>=
)
int num = -8; // 11111111111111111111111111111000 in binary (32-bit)
num >>>= 3; // num is now 536870911 (00011111111111111111111111111111 in binary)
Practical Uses of Bitwise Operators
Bitwise operators are used in various practical tasks such as:
- Cryptography: Encrypting and decrypting data.
- Graphics Programming: Manipulating pixels and colors.
- Network Programming: Managing IP addresses and subnet masks.
- Embedded Systems: Efficiently controlling hardware by setting specific bits.
Bitwise operations are powerful tools for tasks that require low-level data manipulation, often providing speed and efficiency advantages.
19. What are the differences between for
, while
, and do-while
loops in Java?
Loops in Java let you repeat actions several times. The key difference is when the condition is checked.
for – used when you know how many times the loop should run.
public class ForLoopExample { public static void main(String[] args) { for (int i = 0; i < 5; i++) { System.out.println(i); } // Output: 0, 1, 2, 3, 4 } }
while – runs as long as the condition is true. The condition is checked at the start.
public class WhileLoopExample { public static void main(String[] args) { int i = 0; while (i < 5) { System.out.println(i); i++; } // Output: 0, 1, 2, 3, 4 } }
do-while – always runs at least once, even if the condition is false, because the check happens after the loop body.
public class DoWhileLoopExample { public static void main(String[] args) { int i = 0; do { System.out.println(i); i++; } while (i < 5); // Output: 0, 1, 2, 3, 4 } }
In short: a do-while loop will always run at least once.
20. What is a loop iteration?
A loop iteration is a single execution of the code block inside a loop. The loop runs multiple iterations as long as its condition remains true.
Example in Java:
public class ForLoopWithIterations { public static void main(String[] args) { for (int i = 0; i < 3; i++) { System.out.println("Iteration " + i); } } }
In this example, the loop runs three times, from 0 to 2. During each iteration, the value of i
is printed.