Alright, so we already know that Strings in Java are immutable—once created, they can’t be changed. If you try to modify a String
, Java actually creates a new String
object instead of changing the existing one. But this raises a big question:
What Happens to the Old String Object?
Let’s say we do something like this:
String str = "Hello";
str = str + " World"; // Creates a new String "Hello World"
At first, str
was pointing to “Hello”, but after concatenation, it now points to “Hello World”. So what happens to “Hello”?
Does It Stay in Memory Forever?
Nope! The old "Hello"
is no longer referenced, so it becomes eligible for garbage collection. Java’s Garbage Collector (GC) will eventually remove it from memory when it decides it’s the right time.
But here’s the catch: the GC does not run immediately. It cleans up memory only when needed to optimize performance. So "Hello"
might still exist for a short while until the GC actually removes it.
How Does Java Store String References?
When we assign a String
to a variable, that variable stores a reference (a kind of memory address) to the actual string object. Let’s visualize it:
String str1 = "Hello";
String str2 = str1;
Here, both str1
and str2
point to the same "Hello"
object in memory. If we now do:
str1 = "Hello World";
str1
now points to a new"Hello World"
object.str2
still points to the original"Hello"
, so it won’t be garbage collected (yet).
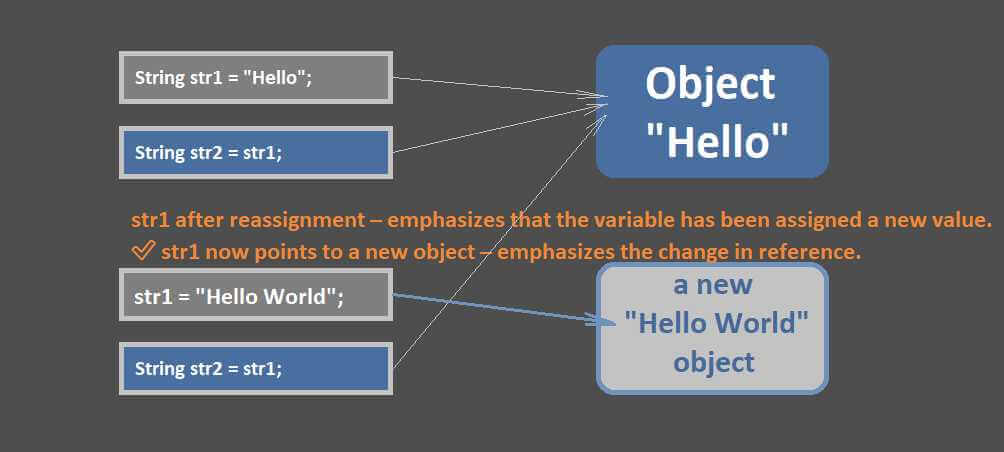
What If We Use new String("Hello")
?
Normally, Java stores string literals in a special memory area called the String Pool to avoid duplication. But if you use new String()
, it forces Java to create a separate object, even if the same string already exists in the pool.
Example:
String str1 = "Hello"; // Stored in String Pool
String str2 = new String("Hello"); // Stored in Heap separately
Here, "Hello"
exists twice in memory—once in the String Pool and once in the Heap. The heap-stored one will be garbage collected when unreferenced.
When Are String References Removed?
A String
reference is removed when there are no more variables pointing to it. For example:
String str = "Hello";
str = null; // Now "Hello" is unreferenced
Since str
now holds null
, the "Hello"
object is ready for garbage collection.
Can Strings Stay in Memory Even If Unused?
Yes! If a String
is stored in the String Pool, it may stay in memory forever (or at least until the JVM shuts down), even if there are no more references to it.
Why?
- The String Pool does not automatically free memory like the heap. Java keeps pooled strings indefinitely to optimize memory usage.
- Interned strings stay in memory even if no references exist outside the pool.
- The JVM only clears the pool when shutting down, meaning those strings may persist as long as the program is running.
Common Misconception: Does intern()
Free Memory?
No, calling “ does NOT remove a string from the pool—it actually moves a string into the pool if it wasn’t there already. This means the string is now part of the JVM’s permanent pool and won’t be garbage collected.
So this line is misleading:
str = str.intern();
Instead of freeing memory, it actually keeps the string in memory longer by ensuring it remains in the pool.
Conclusion
- Strings are immutable, meaning modifications create new objects.
- Old
String
objects become eligible for garbage collection when unreferenced. - String literals are stored in a special String Pool, but
new String()
objects are in the Heap. - The Garbage Collector removes unreferenced strings only when needed, but pooled strings may stay in memory forever.
That’s it!