Welcome to your first programming lesson!
Here’s something exciting to start with: if you’ve decided to learn programming, congratulations—you’re already a programmer! Today, we’re keeping things simple. No overwhelming theory, no intimidating jargon. Instead, we’ll write a basic program together and explore some core concepts.
We’re skipping setting up fancy tools like an IDE (Integrated Development Environment) for now. Instead, let’s begin with something fundamental: data—what it is, where it’s stored, and how it’s processed.
Grab a pencil and some paper; trust me, this will help you:
- Remember things better.
- Practice writing code when you’re away from your computer.
- Prepare for interviews, where you might be asked to write code by hand.
What is data?
Data is any information a program works with—it could be numbers, text, or even more complex things like lists and tables.
To store data, we use something called variables. Think of a variable as a container or a box where we can keep and change data. That’s why they’re called “variables”—their values can vary while the program runs.
Let’s write a program!
Imagine we want our program to calculate the sum of two numbers: 5 and 2. Here’s how we do it:
First, we create a variable to store the number 5:
int x; // Declaring the variable
x = 5; // Assigning a value
To save time, we often combine these steps into one:
int x = 5; // Declaring and initializing the variable in a single line
Now it’s your turn! Create another variable for the number 2. Write a line of code that declares a variable y
of type int
and initializes it with the value 2.
The code for the variable “y” is:
int y = 2; // Declaring and initializing the variable y with the value 2
Next step: storing the result
We need somewhere to save the result of adding x
and y
. Let’s create another variable for that:
int sum = x + y;
Great! You’ve written the core of your program. Let’s see it in action.
Testing your program
Head over to an Online IDE and type in your code (including the variable declarations) above the line:
System.out.println(“Welcome to Online IDE!! Happy Coding :)”);
Then replace:
System.out.println(“Welcome to Online IDE!! Happy Coding :)”);
with this:
System.out.println(“The sum of x and y is: ” + sum);
Like this:
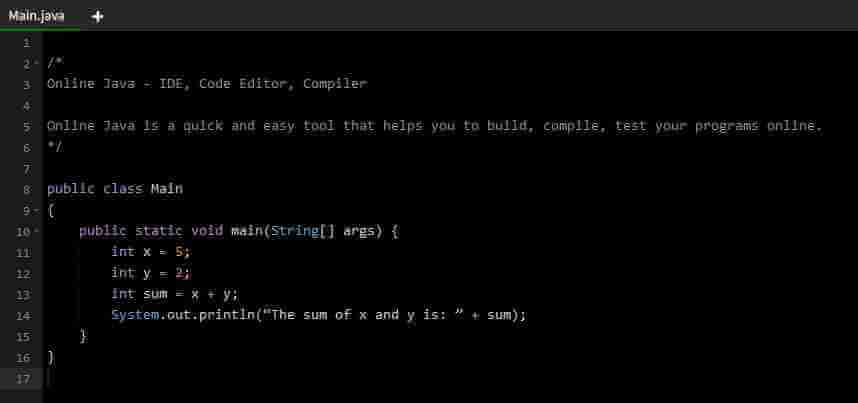
Now, hit Run and watch the magic happen! Try changing the values of x
and y
and run the program again. Notice how the result changes?
This is the meaning of the name “variables”: they store data that your program can work with dynamically, meaning the data in these variables can be changed.
Congratulations!
You’ve just written your first program! It’s a small but important step on your programming journey. Remember, every great developer started exactly where you are today—with curiosity and determination.
What’s next? In the future, we’ll learn about other cool things, like constants and how they differ from variables. But for now, celebrate—you’re officially a programmer! 🎉